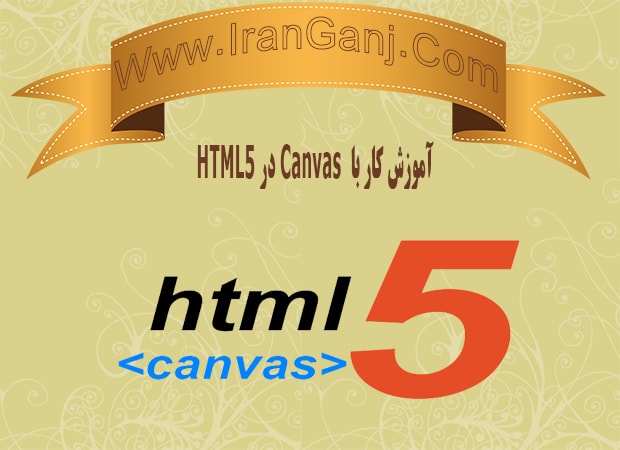
0
0


آموزش کار با Canvas در HTML5 قسمت پنجم
آموزش کار با Canvas در HTML5 قسمت پنجم
در این قسمت از آموزش کار با Canvas به بررسی چند متد دیگر از توابع دو بعدی خواهیم پرداخت.
متد arcTo(x0, y0, x1, y1, RADIUS)
متد arcTo() ترکیبی از متد lineTo() و arc() می باشد.
X0 و y0 یک خط رسم می کند و X1 و y1 یک انحنا با شعاعی RADIUS که مشخص می کنیم که بعد از رسم خط در انتهای آن رسم می کند.
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title></title> <style type="text/css"> canvas { background-color: #fff; } </style> </head> <body> <div> <canvas width="600" height="600" id="canvas"></canvas> </div> <script type="text/javascript" language="javascript"> var canvas = document.getElementById('canvas'); var c = canvas.getContext('2d'); c.beginPath(); c.strokeStyle = "red"; c.moveTo(100, 100); c.lineTo(300, 500); c.lineTo(600, 100); c.lineTo(800, 500); c.lineTo(300, 500); c.stroke(); c.beginPath(); c.strokeStyle = "blue"; c.lineWidth = 5; c.moveTo(100, 100); c.arcTo(300, 500, 600, 100, 120); //c.arcTo(x0, y0, x1, y1, RADIUS); //c.arcTo(600, 100, 800, 500, 50); //c.arcTo(800, 500, 300, 500, 70); c.stroke(); </script> </body> </html>
خروجی
رسم یک آدمک
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title></title> <style type="text/css"> canvas { background-color: #fff; } </style> </head> <body> <div> <canvas width="600" height="600" id="canvas"></canvas> </div> <script type="text/javascript" language="javascript"> var canvas = document.getElementById('canvas'); var c = canvas.getContext('2d'); c.beginPath(); c.arc(200, 200, 50, 0, Math.PI * 2); c.stroke(); c.beginPath(); c.arc(220, 180, 5, 0, Math.PI * 2); c.stroke(); c.beginPath(); c.arc(185, 180, 5, 0, Math.PI * 2); c.stroke(); c.beginPath(); c.arc(200, 210, 25, 0, Math.PI); c.stroke(); c.beginPath(); c.moveTo(200, 250); c.lineTo(200, 500); c.stroke(); c.beginPath(); c.moveTo(200, 300); c.lineTo(300, 400); c.stroke(); c.beginPath(); c.moveTo(200, 300); c.lineTo(100, 400); c.stroke(); </script> </body> </html>
خروجی
رسم یک مربع گوشه گرد با متد arcTo()
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title></title> <style type="text/css"> canvas { background-color: #fff; } </style> </head> <body> <div> <canvas width="700" height="700" id="canvas"></canvas> </div> <script type="text/javascript" language="javascript"> var canvas = document.getElementById('canvas'); var c = canvas.getContext('2d'); var r = 180; c.beginPath(); c.moveTo(100, 100); c.strokeStyle = "red"; c.lineTo(100, 100); c.lineTo(600, 100); c.lineTo(600, 600); c.lineTo(100, 600); c.lineTo(100, 100); c.stroke(); c.beginPath(); c.strokeStyle = "blue"; c.lineWidth = 15; c.moveTo(400, 100); c.arcTo(600, 100, 600, 600, r); c.arcTo(600, 600, 100, 600, r); c.arcTo(100, 600, 100, 100, r); c.arcTo(100, 100, 600, 100, r); c.closePath(); c.stroke(); </script> </body> </html>
خروجی
نکته مهمی از متد arcTo() هنگام رسم منحنی
زمانی که یک منحنی رسم می کنیم نقطه شروع، تماسی به منحنی نداشته باشد (یعنی شعاع دایره بیشتر باشد) خود منحنی نقطه اتصال را تا جای که نیاز باشد در همان راستا ادامه می دهد و سپس منحنی را رسم می کند.
مثال از رسم منحی
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title></title> <style type="text/css"> canvas { background-color: #fff; } </style> </head> <body> <div> <canvas width="600" height="600" id="canvas"></canvas> </div> <script type="text/javascript" language="javascript"> var canvas = document.getElementById('canvas'); var c = canvas.getContext('2d'); c.beginPath(); c.strokeStyle = "red"; c.lineWidth = 8; c.moveTo(200, 200); c.lineTo(300, 100); c.lineTo(500, 300); c.stroke(); c.beginPath(); c.strokeStyle = "green"; c.lineWidth = 4; c.moveTo(200, 200); c.arcTo(300, 100, 700, 400, 300); c.stroke(); </script> </body> </html>
خروجی
رسم یک کمان با Canvas
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title></title> <style type="text/css"> canvas { background-color: #fff; } </style> </head> <body> <div> <canvas width="600" height="600" id="canvas"></canvas> </div> <script type="text/javascript" language="javascript"> var canvas = document.getElementById('canvas'); var c = canvas.getContext('2d'); c.beginPath(); c.strokeStyle = "red"; c.fillStyle = "blue"; c.moveTo(150, 400); c.lineTo(400, 400); c.lineTo(375, 375); c.arcTo(400, 400, 375, 425, 50); c.lineTo(400, 400); c.fill(); c.stroke(); </script> </body> </html>
خروجی
نظر / سوال